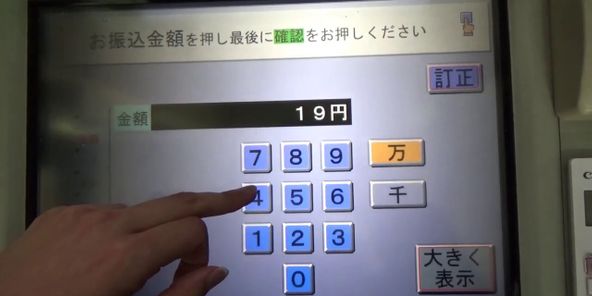
暇なので、pythonを勉強しています。
まず、YoBitからALISの価額を取得しました。
次に、AvacusからもALISの価額を取得し、YoBitと比較するようにしました。
皆さんが思う、主要な仮想通貨はどれでしょう。
BTC、ETH、XRP、XEM、BCH、そしてALISの各価額を、Zaif、YoBit、Avacus、Binance、CREX24という5つの取引所(Avacusは本稿では広義の取引所としておきます)から取得して比較・表示するツールをpythonで作りました。
#
# mccv.py - 主要通貨ビューア
# BTC, ETH, XRP, XEM, BCH, ALISの最新価額(単位:円)を表示する。
# 取得する取引所はZaif, YoBit, Avacus, Binance, CREX24である。
#
import yobit_api
from bs4 import BeautifulSoup
import urllib.request as req
print("■計算の基となる情報")
# YahooファイナンスからUSD/JPYを取得する。
url = "https://stocks.finance.yahoo.co.jp/stocks/detail/?code=usdjpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
usd_jpy = float(soup.select_one(".stoksPrice").string)
print("USD/JPY=", usd_jpy, "(YoBitの価額計算に使われます)")
# YahooファイナンスからEUR/JPYを取得する。
url = "https://stocks.finance.yahoo.co.jp/stocks/detail/?code=eurjpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
eur_jpy = float(soup.select_one(".stoksPrice").string)
print("EUR/JPY=", eur_jpy, "(Binanceの価額計算に使われます)")
print("CREX24は多少の誤差があります。このプログラムではPerfect Money USDをUSDとして扱っているためです。")
print("")
print("■主要通貨情報")
print("取引所 BTC ETH XRP XEM BCH ALIS ")
print("-------------------------------------------------------------------------------------------")
# ====
# Zaif
# ====
Zaif = ["-", "-", "-", "-", "-", "-"]
#BTC/JPY
url = "https://api.zaif.jp/api/1/last_price/btc_jpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Zaif[0] = json["last_price"]
#BTC/JPY
url = "https://api.zaif.jp/api/1/last_price/eth_jpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Zaif[1] = json["last_price"]
#XRP/JPY
#XEM/JPY
url = "https://api.zaif.jp/api/1/last_price/xem_jpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Zaif[3] = json["last_price"]
#BCH/JPY
url = "https://api.zaif.jp/api/1/last_price/bch_jpy"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Zaif[4] = json["last_price"]
#ALIS/JPY
# Zaifの値を表示する。
print("%(ex)7s" %{"ex":"Zaif"}, end = " ")
for i in range(6):
if (Zaif[i] == "-"):
print("%(price)12s " %{"price":"-"}, end = " ")
else:
print("%(price)12.4f " %{"price":Zaif[i]}, end = " ")
print("")
# =====
# YoBit
# =====
YoBit = ["-", "-", "-", "-", "-", "-"]
#BTC/JPY
res = yobit_api.PublicApi().get_pair_ticker(pair="btc_usd")
btc_usd = res.get("last") # 最新の約定価格
btc_jpy= btc_usd * usd_jpy
YoBit[0] = btc_jpy
#ETH/JPY
res = yobit_api.PublicApi().get_pair_ticker(pair="eth_usd")
eth_usd = res.get("last") # 最新の約定価格
eth_jpy= eth_usd * usd_jpy
YoBit[1] = eth_jpy
#XRP/JPY
res = yobit_api.PublicApi().get_pair_ticker(pair="xrp_usd")
xrp_usd = res.get("last") # 最新の約定価格
xrp_jpy= xrp_usd * usd_jpy
YoBit[2] = xrp_jpy
#XEM/JPY
res = yobit_api.PublicApi().get_pair_ticker(pair="xem_usd")
xem_usd = res.get("last") # 最新の約定価格
xem_jpy= xem_usd * usd_jpy
YoBit[3] = xem_jpy
#BCH/JPY
#XALIS/JPY
res = yobit_api.PublicApi().get_pair_ticker(pair="xalis_usd")
xalis_usd = res.get("last") # 最新の約定価格
xalis_jpy= xalis_usd * usd_jpy
YoBit[5] = xalis_jpy
# YoBitの値を表示する。
print("%(ex)7s" %{"ex":"YoBit"}, end = " ")
for i in range(6):
if (YoBit[i] == "-"):
print("%(price)12s " %{"price":"-"}, end = " ")
else:
print("%(price)12.4f " %{"price":YoBit[i]}, end = " ")
print("")
# ======
# Avacus
# ======
Avacus = ["-", "-", "-", "-", "-", "-"]
# BTC/JPY
url = "https://apis.avacus.io/2/market/rates?fiat=jpy&currency=btc"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Avacus[0] = json["data"][0]["btc_jpy"]
# ETH/JPY
# XRP/JPY
# XEM/JPY
# BCH/JPY
url = "https://apis.avacus.io/2/market/rates?fiat=jpy&currency=bch"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Avacus[4] = json["data"][0]["bch_jpy"]
# ALIS/JPY
url = "https://apis.avacus.io/2/market/rates?fiat=jpy&currency=alis"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
Avacus[5] = json["data"][0]["alis_jpy"]
# Avacusの値を表示する。
print("%(ex)7s" %{"ex":"Avacus"}, end = " ")
for i in range(6):
if (Avacus[i] == "-"):
print("%(price)12s " %{"price":"-"}, end = " ")
else:
print("%(price)12.4f " %{"price":Avacus[i]}, end = " ")
print("")
# =======
# Binance
# =======
Binance = ["-", "-", "-", "-", "-", "-"]
# BTC/JPY
url = "https://api.binance.com/api/v3/ticker/price"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
btc_eur = json[695]["price"]
Binance[0] = float(btc_eur) * eur_jpy
# ETH/JPY
eth_eur = json[696]["price"]
Binance[1] = float(eth_eur) * eur_jpy
# XRP/JPY
xrp_eur = json[698]["price"]
Binance[2] = float(xrp_eur) * eur_jpy
# XEM/JPY
xrp_btc = json[282]["price"]
Binance[3] = float(xrp_btc) * Binance[0] # ユーロ建てはないので、BTC建てから円建てに変換
# BCH/JPY
bch_btc = json[666]["price"]
Binance[4] = float(bch_btc) * Binance[0] # ユーロ建てはないので、BTC建てから円建てに変換
# ALIS/JPY
# Binanceの値を表示する。
print("%(ex)7s" %{"ex":"Binance"}, end = " ")
for i in range(6):
if (Binance[i] == "-"):
print("%(price)12s " %{"price":"-"}, end = " ")
else:
print("%(price)12.4f " %{"price":Binance[i]}, end = " ")
print("")
# ======
# CREX24
# ======
CREX24 = ["-", "-", "-", "-", "-", "-"]
# BTC/JPY
url = "https://api.crex24.com/v2/public/tickers?instrument=BTC-USDPM,ETH-USDPM,XRP-BTC,XEM-BTC,BCH-BTC"
res = req.urlopen(url)
soup = BeautifulSoup(res, "html.parser")
json = eval(soup.string)
CREX24[0] = float(json[1]["last"]) * usd_jpy
# ETH/JPY
CREX24[1] = float(json[2]["last"]) * usd_jpy
# XRP/JPY
CREX24[2] = float(json[4]["last"]) * CREX24[0] # BTC建てから円建てに変換
# XEM/JPY
CREX24[3] = float(json[3]["last"]) * CREX24[0] # BTC建てから円建てに変換
# BCH/JPY
CREX24[4] = float(json[0]["last"]) * CREX24[0] # BTC建てから円建てに変換
# ALIS/JPY
# CREX24の値を表示する。
print("%(ex)7s" %{"ex":"CREX24"}, end = " ")
for i in range(6):
if (CREX24[i] == "-"):
print("%(price)12s " %{"price":"-"}, end = " ")
else:
print("%(price)12.4f " %{"price":CREX24[i]}, end = " ")
print("")
長くて難しそうに見えるかもしれませんが、通貨と取引所の組み合わせが多いだけで、1つ1つはたいしたことありません。ソース中の全角「&」は半角に変換してください。
BinanceのAPIは今一つで、通貨ペアごとに値が取れず、全ペアがひとまとまりとなって取れてきます。その中から、696(BTC/EUR)とか282(XEM/BTC)とかの番号を指定して欲しい通貨ペアの価格priceを取得します。番号が変わらない保証があるといいんですが、ドキュメントには見当たりませんでした。
Yobitはドル建て、Binanceはユーロ建てで値が取れるので、それぞれ円建てに変換しています。
前稿の課題だった、書式付き表示がわかりました。C言語のprintf()ライクに書けました。
見た感じ、YoBitはどの通貨も安くなることがときどきあります。Zaif、Binanceは高めです。YoBitで買ってZaifに送り、Zaifで売って出金という戦術がとれるんでしょうか。
他にAPIで価額が取れる取引所があったら、教えてください。
2020/08/14 初版
2020/08/15 取引所CREX24を追加。
以上